Remove.bg API Documentation
Remove Background automatically with 1 API call
Explore our API documentation and examples to integrate remove.bg into your application or workflow.
Not a developer? There are plenty ready to use Tools & Apps by remove.bg and our community.Easy to integrate
Our API is a simple HTTP interface with various options:
- Source images:
- Direct uploads or URL reference
- Result images:
- Image file or JSON-encoded data
- Output resolution:
- up to 50 megapixels
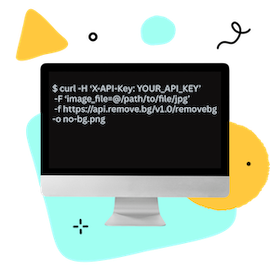
Get started
Our API is a simple HTTP interface with various options:
-
Get your API Key.
Your first 50 API calls per month are on us (see Pricing). - Use the following code samples to get started quickly
- Review the reference docs to adjust any parameters
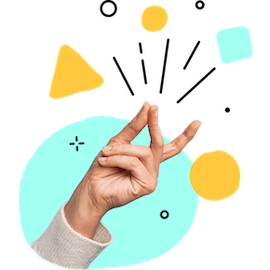
Sample Code
$ curl -H 'X-API-Key: INSERT_YOUR_API_KEY_HERE' \
-F 'image_file=@/path/to/file.jpg' \
-F 'size=auto' \
-f https://api.remove.bg/v1.0/removebg -o no-bg.png
// Requires "axios" and "form-data" to be installed (see https://www.npmjs.com/package/axios and https://www.npmjs.com/package/form-data)
const axios = require('axios');
const FormData = require('form-data');
const fs = require('fs');
const path = require('path');
const inputPath = '/path/to/file.jpg';
const formData = new FormData();
formData.append('size', 'auto');
formData.append('image_file', fs.createReadStream(inputPath), path.basename(inputPath));
axios({
method: 'post',
url: 'https://api.remove.bg/v1.0/removebg',
data: formData,
responseType: 'arraybuffer',
headers: {
...formData.getHeaders(),
'X-Api-Key': 'INSERT_YOUR_API_KEY_HERE',
},
encoding: null
})
.then((response) => {
if(response.status != 200) return console.error('Error:', response.status, response.statusText);
fs.writeFileSync("no-bg.png", response.data);
})
.catch((error) => {
return console.error('Request failed:', error);
});
# Requires "requests" to be installed (see python-requests.org)
import requests
response = requests.post(
'https://api.remove.bg/v1.0/removebg',
files={'image_file': open('/path/to/file.jpg', 'rb')},
data={'size': 'auto'},
headers={'X-Api-Key': 'INSERT_YOUR_API_KEY_HERE'},
)
if response.status_code == requests.codes.ok:
with open('no-bg.png', 'wb') as out:
out.write(response.content)
else:
print("Error:", response.status_code, response.text)
# Install "remove_bg" first (https://github.com/remove-bg/ruby)
require "remove_bg"
RemoveBg.from_file("/path/to/file.jpg",
api_key: "INSERT_YOUR_API_KEY_HERE"
).save("no-bg.png")
// Requires "guzzle" to be installed (see guzzlephp.org)
// If you have problems with our SSL certificate with error 'Uncaught GuzzleHttp\Exception\RequestException: cURL error 60: SSL certificate problem: unable to get local issuer certificate (see https://curl.haxx.se/libcurl/c/libcurl-errors.html) for https://api.remove.bg/v1.0/removebg'
// follow these steps to use the latest cacert certificate for cURL: https://github.com/guzzle/guzzle/issues/1935#issuecomment-371756738
$client = new GuzzleHttp\Client();
$res = $client->post('https://api.remove.bg/v1.0/removebg', [
'multipart' => [
[
'name' => 'image_file',
'contents' => fopen('/path/to/file.jpg', 'r')
],
[
'name' => 'size',
'contents' => 'auto'
]
],
'headers' => [
'X-Api-Key' => 'INSERT_YOUR_API_KEY_HERE'
]
]);
$fp = fopen("no-bg.png", "wb");
fwrite($fp, $res->getBody());
fclose($fp);
// Requires "Apache HttpComponents" to be installed (see hc.apache.org)
Response response = Request.Post("https://api.remove.bg/v1.0/removebg")
.addHeader("X-Api-Key", "INSERT_YOUR_API_KEY_HERE")
.body(
MultipartEntityBuilder.create()
.addBinaryBody("image_file", new File("/path/to/file.jpg"))
.addTextBody("size", "auto")
.build()
).execute();
response.saveContent(new File("no-bg.png"));
// Requires AFNetworking to be installed (see https://github.com/AFNetworking/AFNetworking)
NSURL *fileUrl = [NSBundle.mainBundle URLForResource:@"file" withExtension:@"jpg"];
NSData *data = [NSData dataWithContentsOfURL:fileUrl];
if (!data) {
return;
}
AFHTTPSessionManager *manager =
[[AFHTTPSessionManager alloc] initWithSessionConfiguration:
NSURLSessionConfiguration.defaultSessionConfiguration];
manager.responseSerializer = [AFImageResponseSerializer serializer];
[manager.requestSerializer setValue:@"INSERT_YOUR_API_KEY_HERE"
forHTTPHeaderField:@"X-Api-Key"];
NSURLSessionDataTask *dataTask = [manager
POST:@"https://api.remove.bg/v1.0/removebg"
parameters:nil
constructingBodyWithBlock:^(id _Nonnull formData) {
[formData appendPartWithFileData:data
name:@"image_file"
fileName:@"file.jpg"
mimeType:@"image/jpeg"];
}
progress:nil
success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
if ([responseObject isKindOfClass:UIImage.class] == false) {
return;
}
self.imageView.image = responseObject;
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
// Handle error here
}];
[dataTask resume];
// Requires Alamofire 5 to be installed (see https://github.com/Alamofire/Alamofire)
guard let fileUrl = Bundle.main.url(forResource: "file", withExtension: "jpg"),
let data = try? Data(contentsOf: fileUrl)
else { return false }
struct HTTPBinResponse: Decodable { let url: String }
AF.upload(
multipartFormData: { builder in
builder.append(
data,
withName: "image_file",
fileName: "file.jpg",
mimeType: "image/jpeg"
)
},
to: URL(string: "https://api.remove.bg/v1.0/removebg")!,
headers: [
"X-Api-Key": "INSERT_YOUR_API_KEY_HERE"
]
).responseDecodable(of: HTTPBinResponse.self) { imageResponse in
guard let imageData = imageResponse.data,
let image = UIImage(data: imageData)
else { return }
self.imageView.image = image
}
using (var client = new HttpClient())
using (var formData = new MultipartFormDataContent())
{
formData.Headers.Add("X-Api-Key", "INSERT_YOUR_API_KEY_HERE");
formData.Add(new ByteArrayContent(File.ReadAllBytes("/path/to/file.jpg")), "image_file", "file.jpg");
formData.Add(new StringContent("auto"), "size");
var response = client.PostAsync("https://api.remove.bg/v1.0/removebg", formData).Result;
if(response.IsSuccessStatusCode) {
FileStream fileStream = new FileStream("no-bg.png", FileMode.Create, FileAccess.Write, FileShare.None);
response.Content.CopyToAsync(fileStream).ContinueWith((copyTask) =>{ fileStream.Close(); });
} else {
Console.WriteLine("Error: " + response.Content.ReadAsStringAsync().Result);
}
}
💭 happy easter 2022 from the team at remove.bg
🏁 🍇
😀🔤👋🐣!🔤❗️
🍉
$ curl -H 'X-API-Key: INSERT_YOUR_API_KEY_HERE' \
-F 'image_url=https://www.remove.bg/example.jpg' \
-F 'size=auto' \
-f https://api.remove.bg/v1.0/removebg -o no-bg.png
// Requires "axios" and "form-data" to be installed (see https://www.npmjs.com/package/axios and https://www.npmjs.com/package/form-data)
const axios = require('axios');
const FormData = require('form-data');
const fs = require('fs');
const formData = new FormData();
formData.append('size', 'auto');
formData.append('image_url', 'https://www.remove.bg/example.jpg');
axios({
method: 'post',
url: 'https://api.remove.bg/v1.0/removebg',
data: formData,
responseType: 'arraybuffer',
headers: {
...formData.getHeaders(),
'X-Api-Key': 'INSERT_YOUR_API_KEY_HERE',
},
encoding: null
})
.then((response) => {
if(response.status != 200) return console.error('Error:', response.status, response.statusText);
fs.writeFileSync("no-bg.png", response.data);
})
.catch((error) => {
return console.error('Request failed:', error);
});
# Requires "requests" to be installed (see python-requests.org)
import requests
response = requests.post(
'https://api.remove.bg/v1.0/removebg',
data={
'image_url': 'https://www.remove.bg/example.jpg',
'size': 'auto'
},
headers={'X-Api-Key': 'INSERT_YOUR_API_KEY_HERE'},
)
if response.status_code == requests.codes.ok:
with open('no-bg.png', 'wb') as out:
out.write(response.content)
else:
print("Error:", response.status_code, response.text)
# Install "remove_bg" first (https://github.com/remove-bg/ruby)
require "remove_bg"
RemoveBg.from_url("https://www.remove.bg/example.jpg",
api_key: "INSERT_YOUR_API_KEY_HERE"
).save("no-bg.png")
// Requires "guzzle" to be installed (see guzzlephp.org)
// If you have problems with our SSL certificate getting the error 'Uncaught GuzzleHttp\Exception\RequestException: cURL error 60: SSL certificate problem: unable to get local issuer certificate (see https://curl.haxx.se/libcurl/c/libcurl-errors.html) for https://api.remove.bg/v1.0/removebg'
// follow these steps to use the latest cacert certificate for cURL: https://github.com/guzzle/guzzle/issues/1935#issuecomment-371756738
$client = new GuzzleHttp\Client();
$res = $client->post('https://api.remove.bg/v1.0/removebg', [
'multipart' => [
[
'name' => 'image_url',
'contents' => 'https://www.remove.bg/example.jpg'
],
[
'name' => 'size',
'contents' => 'auto'
]
],
'headers' => [
'X-Api-Key' => 'INSERT_YOUR_API_KEY_HERE'
]
]);
$fp = fopen("no-bg.png", "wb");
fwrite($fp, $res->getBody());
fclose($fp);
// Requires "Apache HttpComponents" to be installed (see hc.apache.org)
Response response = Request.Post("https://api.remove.bg/v1.0/removebg")
.addHeader("X-Api-Key", "INSERT_YOUR_API_KEY_HERE")
.body(
MultipartEntityBuilder.create()
.addTextBody("image_url", "https://www.remove.bg/example.jpg")
.addTextBody("size", "auto")
.build()
).execute();
response.saveContent(new File("no-bg.png"));
// Requires AFNetworking to be installed (see https://github.com/AFNetworking/AFNetworking)
AFHTTPSessionManager *manager =
[[AFHTTPSessionManager alloc] initWithSessionConfiguration:
NSURLSessionConfiguration.defaultSessionConfiguration];
manager.responseSerializer = [AFImageResponseSerializer serializer];
[manager.requestSerializer setValue:@"INSERT_YOUR_API_KEY_HERE"
forHTTPHeaderField:@"X-Api-Key"];
NSURLSessionDataTask *dataTask = [manager
POST:@"https://api.remove.bg/v1.0/removebg"
parameters:@{@"image_url": @"https://www.remove.bg/example.jpg"}
progress:nil
success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
if ([responseObject isKindOfClass:UIImage.class] == false) {
return;
}
self.imageView.image = responseObject;
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"ERROR");
}];
[dataTask resume];
// Requires Alamofire 5 to be installed (see https://github.com/Alamofire/Alamofire)
AF.request(
URL(string: "https://api.remove.bg/v1.0/removebg")!,
method: .post,
parameters: ["image_url": "https://www.remove.bg/example.jpg"],
encoding: URLEncoding(),
headers: [
"X-Api-Key": "INSERT_YOUR_API_KEY_HERE"
]
)
.responseData { imageResponse in
guard let imageData = imageResponse.data,
let image = UIImage(data: imageData)
else { return }
self.imageView.image = image
}
using (var client = new HttpClient())
using (var formData = new MultipartFormDataContent())
{
formData.Headers.Add("X-Api-Key", "INSERT_YOUR_API_KEY_HERE");
formData.Add(new StringContent("https://www.remove.bg/example.jpg"), "image_url");
formData.Add(new StringContent("auto"), "size");
var response = client.PostAsync("https://api.remove.bg/v1.0/removebg", formData).Result;
if(response.IsSuccessStatusCode) {
FileStream fileStream = new FileStream("no-bg.png", FileMode.Create, FileAccess.Write, FileShare.None);
response.Content.CopyToAsync(fileStream).ContinueWith((copyTask) =>{ fileStream.Close(); });
} else {
Console.WriteLine("Error: " + response.Content.ReadAsStringAsync().Result);
}
}
💭 happy easter 2022 from the team at remove.bg
🏁 🍇
😀🔤👋🐣!🔤❗️
🍉
Libraries
Get up and running faster with official and third-party libraries.
Not a developer? There are plenty ready to use Tools & Apps by remove.bg and our community.Output formats
You can request one of three formats via the format
parameter:
Format | Resolution | Pros and cons | Example |
---|---|---|---|
PNG |
Up to 10 Megapixels e.g. 4000x2500 |
+ Simple integration + Supports transparency - Large file size |
Download 7 MB |
JPG |
Up to 50 Megapixels e.g. 8000x62500 |
+ Simple Integration + Small file size - No transparency supported |
Download 1 MB |
ZIP |
Up to 50 Megapixels e.g. 8000x6250 |
+ Small file size + Supports transparency - Integration requires compositing |
Download 3 MB |
Please note that PNG images above 10 megapixels are not supported. If you require transparency for images of that size, use the ZIP format (see below). If you don't need transparency (e.g. white background), we recommend JPG.
How to use the ZIP format
The ZIP format has the best runtime performance for transparent images.
In comparison to PNG, the resulting file is up to 80% smaller (faster to download) and up to 40% faster to generate. For performance optimization we recommend using the ZIP format whenever possible. Above 10 megapixels, usage of the ZIP format is required for transparent results.
The ZIP file always contains the following files:
color.jpg | A non-transparent RGB image in JPG format containing the colors for each pixel. (Note: This image differs from the input image due to edge color corrections.) – Show example |
alpha.png | A non-transparent grayscale image in PNG format containing the alpha matte. White pixels are foreground regions, black is background. – Show example |
To compose the final image file:
- Extract the files from the ZIP
- Apply the alpha matte (alpha.png) to the color image (color.jpg).
- Save the result in a format of your choice (e.g. PNG)
Sample code for linux (requires zip and imagemagick):
$ unzip no-bg.zip && \
convert color.jpg alpha.png \
-compose CopyOpacity \
-composite no-bg.png
A zip2png
command is integrated in our command line interface. More code samples can be found here.
OAuth 2.0
If you want to authenticate users with the click of a button instead of having them enter an API Key, get in touch to try our OAuth 2.0 login.
API Reference
Rate Limit
You can process up to 500 images per minute through the API, depending on the input image resolution in megapixels.
Examples:
Input image | Megapixels | Effective Rate Limit |
---|---|---|
625 x 400 | 1 MP | 500 images per minute |
1200 x 800 | 1 MP | 500 images per minute |
1600 x 1200 | 2 MP | 500 / 2 = 250 images per minute |
2500 x 1600 | 4 MP | 500 / 4 = 125 images per minute |
4000 x 2500 | 10 MP | 500 / 10 = 50 images per minute |
6250 x 4000 | 25 MP | 500 / 25 = 20 images per minute |
8000 x 6250 | 50 MP | 500 / 50 = 10 images per minute |
Exceedance of rate limits leads to a HTTP status 429 response (no credits charged). Clients can use the following response headers to gracefully handle rate limits:
Response header | Sample value | Description |
---|---|---|
X-RateLimit-Limit
|
500
|
Total rate limit in megapixel images |
X-RateLimit-Remaining
|
499
|
Remaining rate limit for this minute |
X-RateLimit-Reset
|
1714205621
|
Unix timestamp when rate limit will reset |
Retry-After
|
59
|
Seconds until rate limit will reset (only present if rate limit exceeded) |
Higher rate limits are only available to high-volume solution users; get in touch here.
Exponential backoff
Exponential backoff is an error handling strategy in which a client periodically retries a failed request.
The delay increases between requests and often includes jitter (randomized delay) to avoid collisions when using concurrent clients.
Clients should use exponential backoff whenever they receive HTTP status codes 5XX
or 429
.
The following pseudo code shows one way to implement exponential backoff:
retry = true
retries = 0
WHILE (retry == TRUE) AND (retries < MAX_RETRIES)
wait_for_seconds (2^retries + random_number)
result = get_result
IF result == SUCCESS
retry = FALSE
ELSE IF result == ERROR
retry = TRUE
ELSE IF result == THROTTLED
retry = TRUE
END
retries = retries +1
END
API Changelog
Most recent API updates:
-
2024-03-17: - Added new value for size parameter:
50MP
for up to 50 megapixels (e.g., 8000x6250 pixels) with formats ZIP or JPG, or up to 10 megapixels (e.g., 4000x2500 pixels) with PNG – costs 1 credit per image. The "auto" option keeps supporting a maximum of 25MP for backwards compatibility. -
2024-01-10: Final reminder - Deprecation of
TLS 1.0
and1.1
. Upgrade toTLS 1.2
or higher for uninterrupted service before 2024-02-05. -
2023-12-06: Second notice - Deprecation of
TLS 1.0
and1.1
. Upgrade toTLS 1.2
or higher for uninterrupted service before 2024-02-05. -
2023-11-22: First notice - Deprecation of
TLS 1.0
and1.1
. Upgrade toTLS 1.2
or higher for uninterrupted service before 2024-02-05. -
2021-12-07: Added foreground position and size to background removal responses. (JSON fields:
foreground_top
,foreground_left
,foreground_width
andforeground_height
. Response headers:X-Foreground-Top
,X-Foreground-Left
,X-Foreground-Width
andX-Foreground-Height
.) -
2021-04-13: Removed deprecated
shadow_method=legacy
option andshadow_method
parameter as it no longer has any effect. -
2021-03-01: Added examples for
400
error codes. -
2021-01-21: Added
shadow_method
parameter to control shadow appearance. Deprecatedlegacy
value forshadow_method
. -
2020-09-30: Added
type_level
parameter andPOST /improve
endpoint. -
2020-05-06: Added
semitransparency
parameter. - 2019-09-27: Introduce ZIP format and support for images up to 25 megapixels.
- 2019-09-25: Increased maximum file size to 12 MB and rate limit to 500 images per minute. Rate limit is now resolution-dependent.
-
2019-09-16: Added
enterprise
credit balance field to account endpoint. -
2019-08-01: Added
add_shadow
option for car images. -
2019-06-26: Added
crop_margin
,scale
andposition
parameters. -
2019-06-19: Added support for animals and other foregrounds (response header
X-Type: animal
andX-Type: other
) -
2019-06-11: Credit balances can now have fractional digits, this affects the
X-Credits-Charged
value -
2019-06-03: Added parameters
bg_image_url
,bg_image_file
(add a background image),crop
(crop off empty regions) androi
(specify a region of interest). -
2019-05-13: Added car support (
type=car
parameter andX-Type: car
response header) -
2019-05-02: Renamed size
"regular"
to"small"
. Clients should use the new value, but the old one will continue to work (deprecated) -
2019-05-01: Added endpoint
GET /account
for credit balance lookups -
2019-04-15: Added parameter
format
to set the result image format -
2019-04-15: Added parameter
bg_color
to add a background color to the result image